Last Updated on January 18, 2025
Want to use PowerShell for generating passwords?
In this guide, you will learn how to generate a random password with PowerShell, along with a few more advanced options.
Let’s get started!
Table of Contents:
Why use PowerShell as a password generator?
We have quite a few password generators these days, and many of them integrate with a lot of software and browsers.
However, there are still a lot of people who are comfortable with using PowerShell for generating passwords.
Here are some of their reasons:
- Flexibility and customization
- Automation capabilities
- Integration and scripting power
Since PowerShell is highly customizable, you can use it to generate passwords that meet specific criteria.
Examples are:
- Length
- Complexity
- Inclusion of various character sets
Since PowerShell is deeply integrated with Windows, you can integrate your scripts into large automation tasks.
A bonus point here is that using PowerShell doesn’t require any user accounts or service accounts.
Sign up for exclusive updates, tips, and strategies
How to Generate Random Passwords
There are cases when all you need is a simple password (where super secure passwords aren’t a concern).
For that, here’s a basic script you can use:
function Generate-SimplePassword {
$length = 8
$characters = 'abcdefghijkmnopqrstuvwxyzABCDEFGHJKLMNPQRSTUVWXYZ23456789'
$password = -join ((1..$length) | ForEach-Object { Get-Random -Maximum $characters.length } | ForEach-Object { $characters[$_]} )
return $password
}
Generate-SimplePassword
This script defines a function called Generate-SimplePassword that:
- Sets the desired password length to 8 characters.
- Specifies a character set that includes uppercase letters, lowercase letters, and numbers (excluding characters that are often confused, like ‘l’, ‘1’, ‘o’, ‘0’).
- Uses a loop to select random characters from the specified set until it reaches the desired length.
- Returns the generated password.
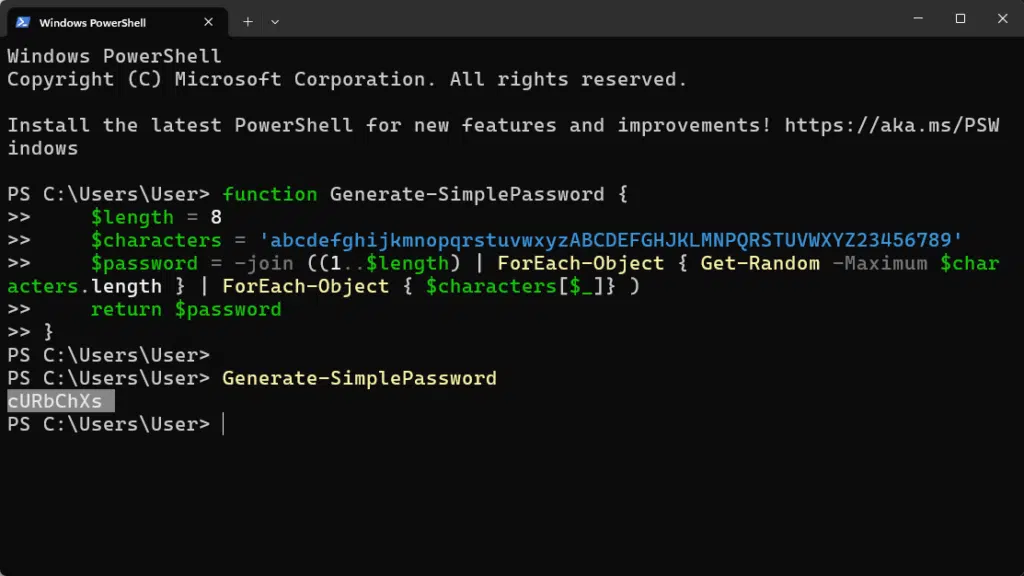
Don’t forget that this script generates a less complex password.
If you need a better one, read on. 🙂
How to Generate a Password with Specific Requirements
But what if you have specific requirements?
Well, let’s say you need at least one number and one special character, below is a script that you can use:
function Generate-ComplexPassword {
param (
[int]$length = 12
)
$upperCase = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
$lowerCase = 'abcdefghijklmnopqrstuvwxyz'
$numbers = '0123456789'
$specialChars = '!@#$%^&*()_-+=<>?'
# Ensure the password includes at least one character from each set
$initialPassword = ($upperCase | Get-Random -Count 1) +
($lowerCase | Get-Random -Count 1) +
($numbers | Get-Random -Count 1) +
($specialChars | Get-Random -Count 1)
$allChars = $upperCase + $lowerCase + $numbers + $specialChars
# Fill the rest of the password up to the desired length
for ($i = $initialPassword.Length; $i -lt $length; $i++) {
$initialPassword += $allChars | Get-Random -Count 1
}
# Shuffle the password to prevent predictable patterns
$shuffledPasswordChars = $initialPassword.ToCharArray() | Get-Random -Count $length
$shuffledPassword = -join $shuffledPasswordChars
return $shuffledPassword
}
Generate-ComplexPassword -length 12
This script defines a function called Generate-ComplexPassword which:
- Accepts a length parameter with a default value of 12 characters.
- Defines character sets for uppercase letters, lowercase letters, numbers, and special characters.
- Ensures the generated password starts with at least one character from each set to meet the specified requirements.
- Fills the rest of the password length with random characters from the combined set.
- Shuffles the password characters to remove any predictable sequence, enhancing security.
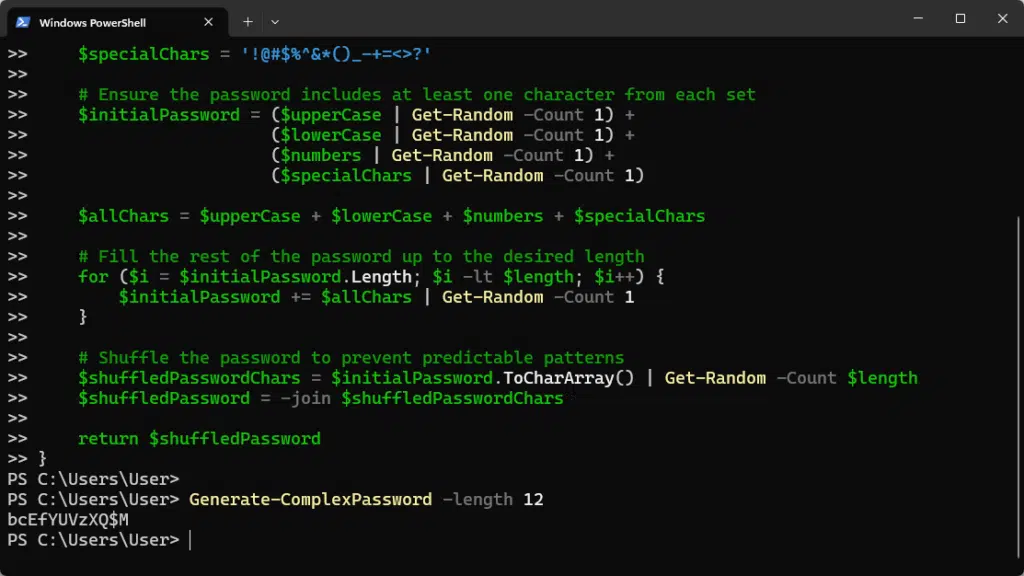
You can adjust the length parameter as needed while still meeting your complexity requirements.
How to Generate a Strong Password with PowerShell
The section above can already generate a pretty strong password.
But you can increase the complexity and length of the password (like including a broader range of characters).
Here’s a sample script:
function Generate-StrongPassword {
param (
[int]$length = 16 # Increase length for stronger passwords
)
# Character sets
$upperCase = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
$lowerCase = 'abcdefghijklmnopqrstuvwxyz'
$numbers = '0123456789'
$specialChars = '!@#$%^&*()_-+=<>?:{}[]|~`'
# Ensure at least one of each type is included
$passwordChars = @(
($upperCase.ToCharArray() | Get-Random),
($lowerCase.ToCharArray() | Get-Random),
($numbers.ToCharArray() | Get-Random),
($specialChars.ToCharArray() | Get-Random)
)
# Available character sets array
$allCharSets = @($upperCase, $lowerCase, $numbers, $specialChars)
# Combine all characters into a single string for further character selection
$allChars = $upperCase + $lowerCase + $numbers + $specialChars
# Fill the rest of the password
for ($i = $passwordChars.Count; $i -lt $length; $i++) {
$passwordChars += $allChars.ToCharArray() | Get-Random
}
# Shuffle the password characters to avoid any patterns
$shuffledPasswordChars = $passwordChars | Get-Random -Count $length
$strongPassword = -join $shuffledPasswordChars
return $strongPassword
}
Generate-StrongPassword -length 16
That’s a lot of text — but it basically includes a broader range like uppercase and lowercase letters, special characters, etc.
Here’s the result:
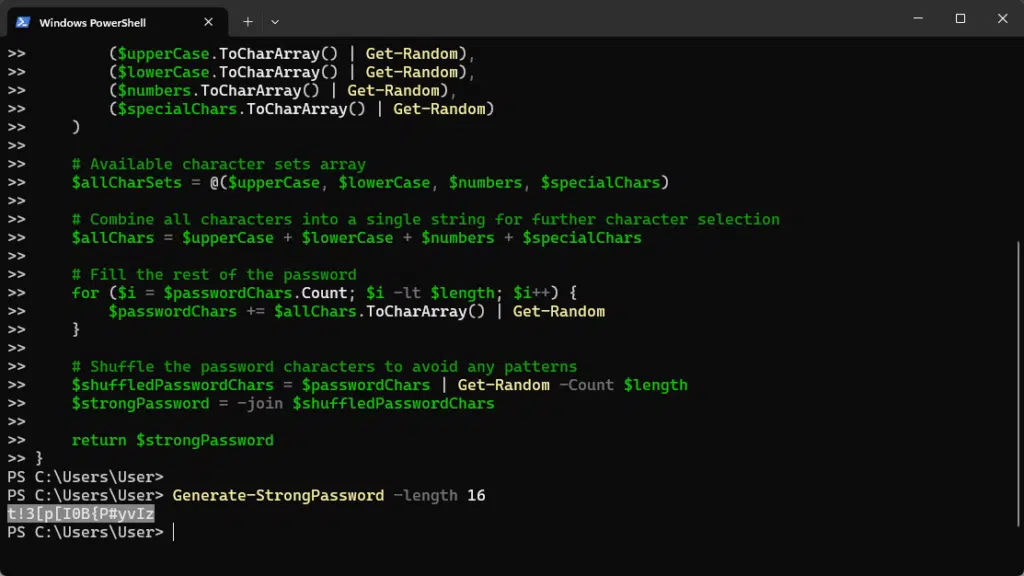
This introduces a randomness factor in the selection of character types for each position to make it less predictable.
Anyway, do you have any questions about using PowerShell for password generation? Leave a comment below.
For business-related questions and concerns, you can always reach me using the contact form. I’ll reply asap.